ESC
Components > Prompt Input
YourComponent.vue
1import { PromptInput } from "@/components/app-components";
A complete and customizable prompt input component that lets you take user input, adjust the strength of different parts of the prompt, and generate images based on the prompt.
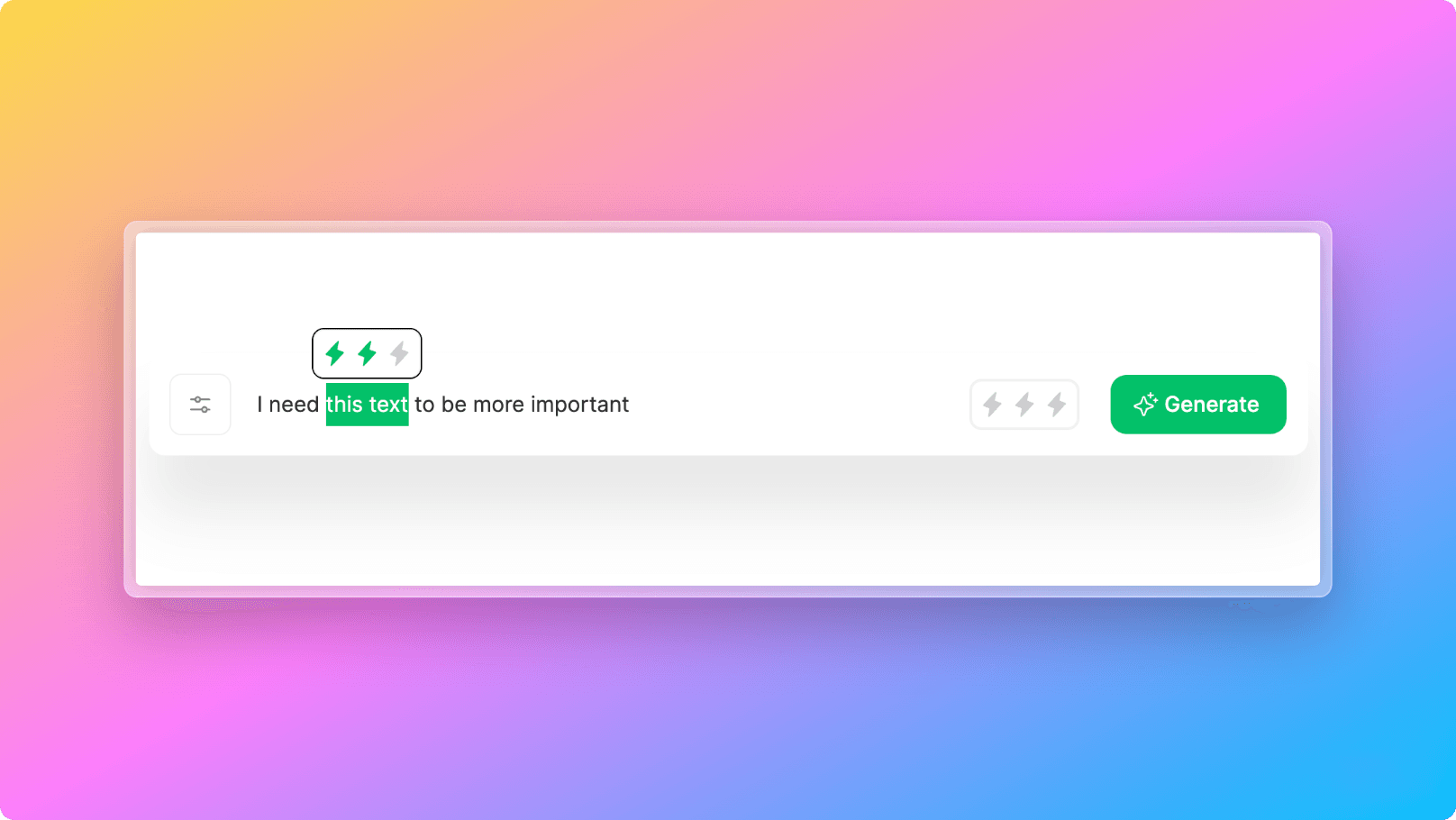
It also supports negative prompts 🤩 Ideal for apps that create images based on user prompts!
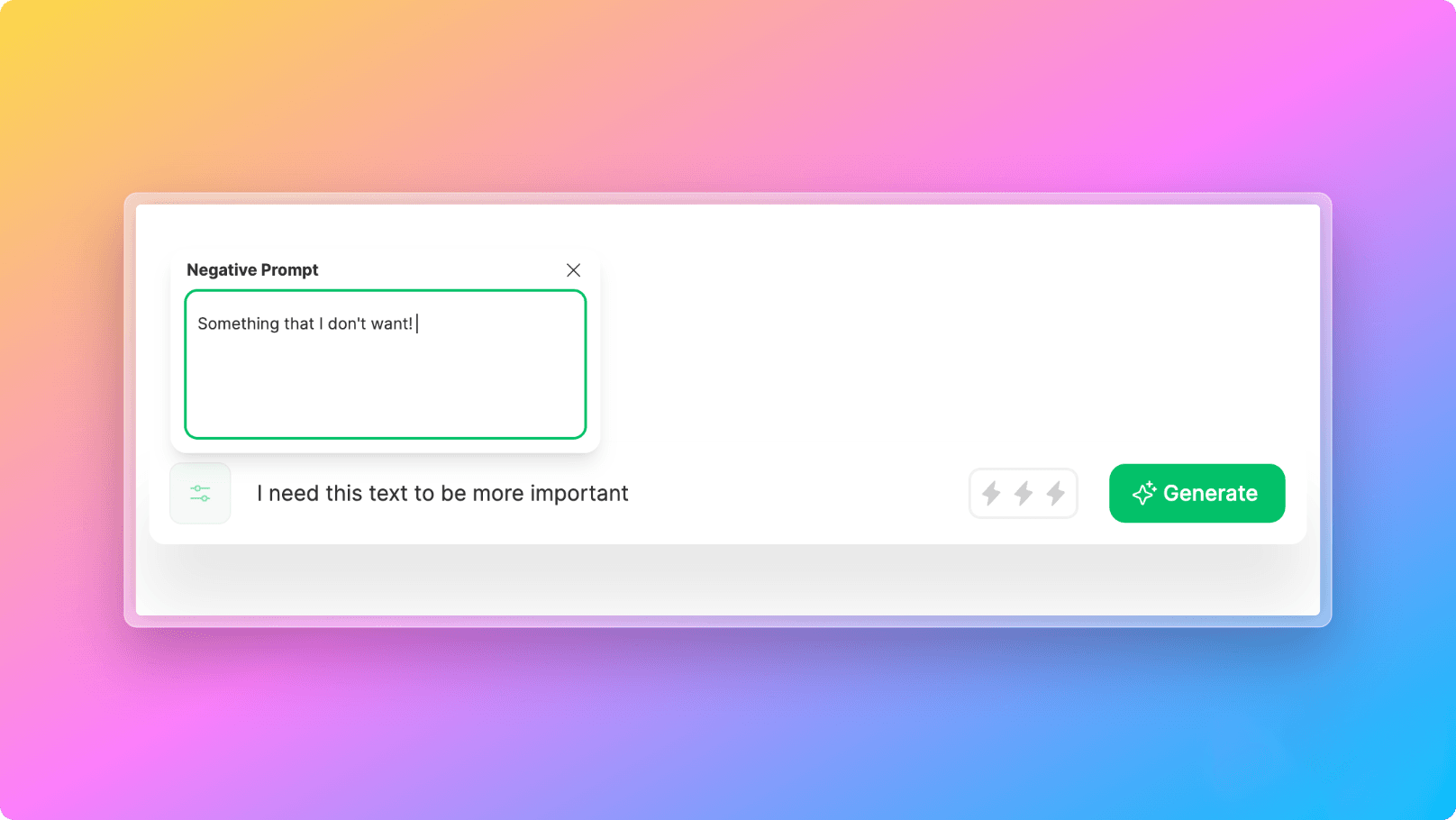
Usage
To use the PromptInput component, you need to control its state from a parent component, managing both the weighted prompts and the negative prompt.
YourCustomComp.vue
1<template>
2 <PromptInput v-model:negative-prompt="negativePrompt" :prompt="prompt" @update:prompts="handleUpdatePrompts"
3 class="max-[567px]:flex-col w-full" @click="handleClick" />
4</template>
5<script setup lang="ts">
6import { PromptInput } from '@/components/app';
7
8const negativePrompt = ref('');
9const prompt = ref('');
10const handleClick = () => alert("Generate clicked");
11const handleUpdatePrompts = (prompts: { text: string; importance: number }[]) => console.log('prompts', prompts);
12</script>
Weighted Prompts Format
The prompts state should be an array of objects, with each object representing a part of the prompt and its associated importance level.
prompts.ts
1
2 [
3 {"text": "text part", "importance": importance level},
4 ...
5 ]
Importance Levels:
- -> 0: Low importance.
- -> 1: Medium importance.
- -> 2: High importance.
Converting it to weighted prompt
Many generative image AI models use a weighted prompt syntax for their input.Ex: dog (barking:1.1) near (a beer:1.3)
You can create a utility function to get the desired output, like the following:
prompt-utils.ts
1
2 function convertWeightedPromptsToString(prompts) {
3 // Map each prompt to a string, adding the importance directly without modification
4 const promptStrings = prompts.map(prompt => {
5 if (prompt.importance === 0) {
6 return prompt.text; // No importance shown for 0
7 }
8 // Adjusting the importance format as per your requirement
9 const adjustedImportance = prompt.importance === 1 ? "1.1" : "1.3";
10 return `(${prompt.text}:${adjustedImportance})`;
11 });
12
13 // Join all prompt strings into one, separating them by spaces
14 return promptStrings.join(" ");
15 }
16
17 // Usage example
18 const weightedPrompts = [
19 { text: "penguin", importance: 0 },
20 { text: "holding", importance: 1 },
21 { text: "a beer", importance: 2 }
22 ];
23
24 const outputString = convertWeightedPromptsToString(weightedPrompts);
25 console.log(outputString);
26 // penguin (holding:1.1) (a beer:1.3)