Components > Canvas
1<script lang="tsx" setup>
2 import { Canvas } from '@/components/app';
3 import { useCanvasProvider } from '@/composables/useCanvasProvider';
4</script>
🎨 Add a flexible and customizable canvas component to your app, which can handle both user-drawn sketches and uploaded images. Users can easily choose from over 1000 icons and SVGs directly within the canvas component. Ideal for creating image AI apps, design apps, and more. This component helps you easily incorporate a wide range of visual input from users into your application.
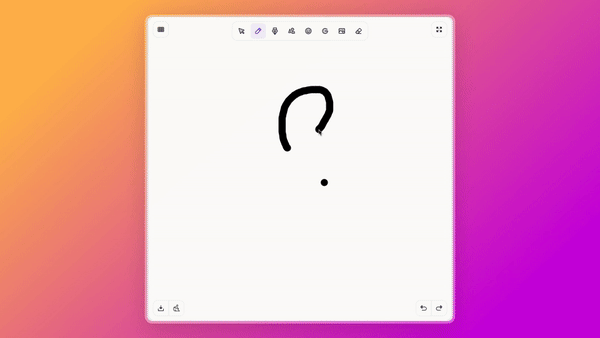
Features
- -> Features for drawing and editing that include support for shapes, icons, brand logos, and personalized images.
- -> Extra options like undo/redo, clear the canvas, download your design, and full-screen view.
- -> Buttons that you can customize to show or hide certain features.
- -> Connected with Vue Provide / Inject for easy access to canvas features and states.
Customizing Canvas
You can customize the Canvas component by changing settings in the configuration object. This lets you choose which overlay buttons are visible based on what your application needs.
1
2 ...
3 canvas: {
4 hideShapes: false, // Hide the shapes button
5 hideIcons: false, // Hide the icons button
6 hideBrands: false, // Hide the brands button
7 hideUpload: false, // Hide the upload button
8 hideEraser: false, // Hide the eraser button
9 hideDownload: false, // Hide the download button
10 hideClear: false, // Hide the clear canvas button
11 hideUndoRedo: false, // Hide the undo/redo buttons
12 hideGrid: false, // Hide the grid button
13 hideFullscreen: false, // Hide the fullscreen button
14 },
15 ...
16
Vue Provide/Inject Usage
With a simple Vue Provide/Inject useCanvasProvider: With many features separate from the overall application logic, everything related to the canvas can be managed by simply importing the canvas context into your application, as shown below:
1import { useCanvasProvider } from '@/composables/useCanvasProvider';
2const {
3 setMenuState, // Setter for active drawing state (select, brush, line, eraser etc.)
4 menuState, // Getter for active drawing state.
5 fabricCanvas, // The instance of the Fabric.js canvas.
6 undo, // Function to undo the last action.
7 redo, // Function to redo the last action.
8 clear, // Function to clear the canvas.
9 download, // Function to download canvas as PNG
10 brushWidth, // Getter for brush size.
11 setBrushWidth, // Setter for brush size.
12 eraserWidth, // Getter for eraser size.
13 setEraserWidth, // Setter for eraser size.
14 addSvg, // Function to add svg images to canvas.
15 addImage, // Function to add base64 encoded images to canvas.
16 addImageUrl, // Function to add remote images to canvas.
17 fullScreen, // Getter for fullscreen state.
18 setFullScreen, // Setter for fullscreen state.
19 addGridBackground, // Setter for grid state.
20 removeGridBackground, // Setter for grid state.
21 gridBackgroundActive, // Getter for grid state.
22 activeSelection, // Getter for active canvas selection.
23 setActiveSelection, // Setter for active canvas selection.
24} = useCanvasProvider();
25
Usage
1<script setup>
2 import { provide } from 'vue';
3 import { Canvas } from '@/components/app';
4 import { useCanvasProvider } from '@/composables/useCanvasProvider';
5
6 const canvasContext = useCanvasProvider();
7 provide('canvasContext', canvasContext);
8
9 const handleWindowSizeChange = () => {
10 const object = document.getElementById("canvas-showcase-wrapper");
11 let containerWidth = 0;
12 let containerHeight = 0;
13 if (canvasContext.fullScreen.value) {
14 containerWidth = window.innerWidth;
15 containerHeight = window.innerHeight;
16 } else if (object.clientWidth > 600) {
17 containerWidth = 600 * 0.87;
18 containerHeight = 600 * 0.87;
19 } else {
20 containerWidth = object.clientWidth * 0.87;
21 containerHeight = object.clientHeight * 0.87;
22 }
23
24 const min = Math.min(containerWidth, containerHeight);
25
26 const scale = min / canvasContext.fabricCanvas.value.getWidth();
27
28 if (scale === 1) return;
29 const zoom = canvasContext.fabricCanvas.value.getZoom() * scale;
30 canvasContext.fabricCanvas.value.setDimensions({
31 width: min,
32 height: min,
33 });
34 canvasContext.fabricCanvas.value.setViewportTransform([zoom, 0, 0, zoom, 0, 0]);
35 };
36
37 watch(() => canvasContext.fullScreen.value, () => {
38 handleWindowSizeChange();
39 }, {})
40
41 onMounted(() => {
42 canvasContext.setMenuState("Brush");
43 window.addEventListener("resize", handleWindowSizeChange);
44 handleWindowSizeChange();
45 });
46 onUnmounted(() => {
47 window.removeEventListener("resize", handleWindowSizeChange);
48 });
49 </script>